비타그램 미니 종아리 발마사지기 LF-199: 2023년 핫한 발 건강 관리템 분석
추천 상품 순위 정보 |
---|
🔹 1위, 비타그램 미니 종아리 발마사지기 LF-199 |
🔸 게시물 삭제 안내 |
안녕하세요! 꼭 소개하고 싶은
비타그램 미니 종아리 발마사지기 LF-199에 대한 상품 TOP1개 상품을 추천해드리려고 합니다.
👍 판매량이 많은, ⭐ 후기도 좋은, 💰 가성비도 좋은 비타그램 미니 종아리 발마사지기 LF-199 상위 1개 제품 확인해보세요!
클릭하시면 비타그램 미니 종아리 발마사지기 LF-199에 대한 더욱 자세한 제품 정보를 보실 수 있습니다.
📋 비타그램 미니 종아리 발마사지기 LF-199에 대한 상품 1개를 선정해봤어요!.
🔍 제품의 특징을 확인하시고, 🛒 원하시는 제품을 선택해보세요!
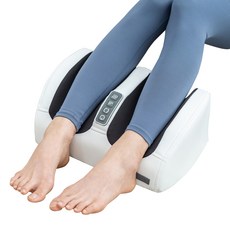
비타그램 미니 종아리 발마사지기 LF-199 인기 후기 Top1 1위
비타그램 미니 종아리 발마사지기 LF-199
79,000원
39,900원
(49%)
⭐ 4.5점 | 💬 1539개
로켓배송: 🚀가능🚀
🔍 제품의 특징을 알아보아요 🔍
✔️ **휴대하기 간편한 미니 사이즈**: 작고 가벼운 디자인으로 집안 어디든 옮겨 다니며 사용 가능하여 공간 제약 없이 편리하게 마사지를 즐길 수 있습니다.
✔️ **강력한 마사지 기능**: 다양한 마사지 모드와 강도 조절 기능을 통해 종아리와 발의 피로를 효과적으로 해소합니다.
✔️ **합리적인 가격**: 뛰어난 성능과 기능을 갖춘 제품임에도 불구하고 부담 없는 가격으로 누구나 쉽게 사용할 수 있습니다.
이 글은 파트너스 활동으로서 일정 수익을 지급받을수 있습니다!
📧 게시물 삭제 요청 📧
상표권&저작권에 문제가 되는 게시물 삭제에 관하여 메일로 문의를 보내주시면 감사하겠습니다.
💌 메일주소 💌
ghrms6320@naver.com