리빙 & 소품 사진 촬영의 완벽한 도구: 핸드폰 수직 촬영 거치대 분석
추천 상품 순위 정보 |
---|
🔹 1위, 리빙앤소품 항공샷 수직촬영 링라이트 핸드폰 촬영 거치대, 1개 |
🔸 게시물 삭제 안내 |
안녕하세요! 감성을 자극하는
리빙앤소품 항공샷 수직촬영 핸드폰 촬영 거치대, 1개에 대한 상품 TOP1개 상품을 추천해드리려고 합니다.
👍 판매량이 많은, ⭐ 후기도 좋은, 💰 가성비도 좋은 리빙앤소품 항공샷 수직촬영 핸드폰 촬영 거치대, 1개 상위 1개 제품 확인해보세요!
클릭하시면 리빙앤소품 항공샷 수직촬영 핸드폰 촬영 거치대, 1개에 대한 더욱 자세한 제품 정보를 보실 수 있습니다.
📋 리빙앤소품 항공샷 수직촬영 핸드폰 촬영 거치대, 1개에 대한 상품 1개를 선정해봤어요!.
🔍 제품의 특징을 확인하시고, 🛒 원하시는 제품을 선택해보세요!
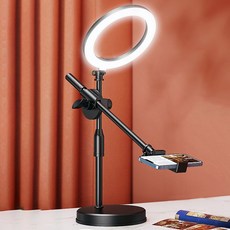
리빙앤소품 항공샷 수직촬영 핸드폰 촬영 거치대, 1개 인기 후기 Top1 1위
리빙앤소품 항공샷 수직촬영 링라이트 핸드폰 촬영 거치대, 1개
17,900원
16,350원
(8%)
⭐ 4.0점 | 💬 16개
로켓배송: 🚀가능🚀
🔍 제품의 특징을 알아보아요 🔍
✔️ 항공샷 및 수직 촬영에 적합한 디자인으로 다양한 각도 연출 가능
✔️ 링라이트 내장으로 밝고 선명한 사진 촬영 가능
✔️ 핸드폰 거치대 기능으로 손쉽게 촬영 환경 구축 가능
위 글은 광고활동으로 일정 수익금을 받을수 있습니다 ^0^
📧 게시물 삭제 요청 📧
상표권&저작권에 문제가 되는 게시물 삭제에 관하여 메일로 문의를 보내주시면 감사하겠습니다.
💌 메일주소 💌
ghrms6320@naver.com